In
mathematics, the
Fibonacci numbers or
Fibonacci series or
Fibonacci sequence are the numbers in the following
integer sequence:

By definition, the first two numbers in the Fibonacci sequence are 0
and 1 (alternatively, 1 and 1), and each subsequent number is the sum of
the previous two.
In mathematical terms, the sequence
Fn of Fibonacci numbers is defined by the
recurrence relation

with seed values
[1]

The Fibonacci sequence is named after
Leonardo of Pisa, who was known as Fibonacci. Fibonacci's 1202 book
Liber Abaci introduced the sequence to Western European mathematics,
[2] although the sequence had been described earlier in
Indian mathematics.
[3][4][5] (By modern convention, the sequence begins either with
F0 = 0 or with
F1 = 1. The
Liber Abaci began the sequence with
F1 = 1, without an initial 0.)
Fibonacci numbers are closely related to
Lucas numbers in that they are a complementary pair of
Lucas sequences. They are intimately connected with the
golden ratio, for example the
closest rational approximations to the ratio are 2/1, 3/2, 5/3, 8/5, ... . Applications include computer algorithms such as the
Fibonacci search technique and the
Fibonacci heap data structure, and graphs called
Fibonacci cubes used for interconnecting parallel and distributed systems. They also appear in biological settings,
[6] such as branching in trees,
phyllotaxis (the arrangement of leaves on a stem), the fruit spouts of a
pineapple,
[7] the flowering of
artichoke, an uncurling
fern and the arrangement of a
pine cone.
[8]
 |
A tiling with squares whose sides are successive Fibonacci numbers in length |
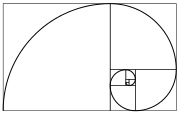 |
A Fibonacci spiral created by drawing circular arcs connecting the
opposite corners of squares in the Fibonacci tiling; this one uses
squares of sizes 1, 1, 2, 3, 5, 8, 13, 21, and 34. |
In simple words we can understand that it start from 1,1 and next number is the sum of last two numbers like
1,1,1+1=2,1+2=3,2+3=5,3+5=8,5+8=13,....
( 1 , 1 , 2 ,3 , 5 , 8 , 13 ,...)
so the main logic behind this problem is two take last two no and add them so the next no can be obtain
so the code :
1. while(i<=n-2)
2.{
3 c=a+b;
4. printf("%d ",c);
5. a=b;
6. b=c;
7. i++;
}
take essential part in this program lets have a look at the code:
Statement 1.This is the starting of loop.it shows that loop will iterate upto and equal to n-2.
Statement 2.This is opening curly brace which shows the starting of loop or any block.
Statement 3.This is the addition operation of last two no.like
(1,1)
(a ,b)
so what will be c =a+b
(1,1,1+1)
(a, b, c )
Statement 4.This is the print function it output next no(c) on the monitor screen
Statement 5 and Statement 6 .In these statement b becomes a and c becomes b like this
(1,1,2,1+2 )
(a ,b,c, d)
(_, b=a',c=b',c'=a'+b'=d)
Statement 7 .finally the increment of the loop variable(i) and end of loop('}')
Code:
//fibonaci series
#include<stdio.h>
#include<conio.h>
void main()
{
clrscr();
int a=1,b=1;
void fibo(int,int);//declaration part of function fibo().
fibo(a,b); //now print rest of the values by return from the function(Calling of fibo()).
getch();//it waits for the user for the input so it holds the input screen until the input is given.
}
void fibo(int a,int b)
{
int n,i=1, c;
printf("enter value of n:",n);
scanf("%d",&n);
printf("%d ,%d ",a,b);
while(i<=n-2)
{
c=a+b;
printf(", %d ",c);
a=b;
b=c;
i++;
}
}
Output :
enter value of n:5
1, 1 ,2, 3, 5
here
red no. (5) is given by user as a input